This docker startup tutorial is designed for everyone who is interested to learn docker in a easy way. Main goal behind this tutorial is to make docker learning simple. As docker has simplified many things for software development, hence it is very important for everyone to know how to use docker in software development.
In this blog we will cover everything you need to get started with docker and skip some technical or theoretical details which are optional to understand in this phase. However, links will be provided where you can read if you are interested to know more.
Introduction
Sometimes setting up the environment for application could be very time consuming and difficult. Everything required to run application has to be setup properly like database, environment variables, required libraries etc. Just imagine how cool it would be if this can be done with single click? Yes, that we can achieve using docker😊.
What is docker?
Docker allows to package the application with required files and environment, share and run the application.
Docker is an opensource project for automation of most stages of the software lifecycle. For eg., packaging, sharing and deploying the application with required settings.
We can combine everything into a package then we can share or run it directly.
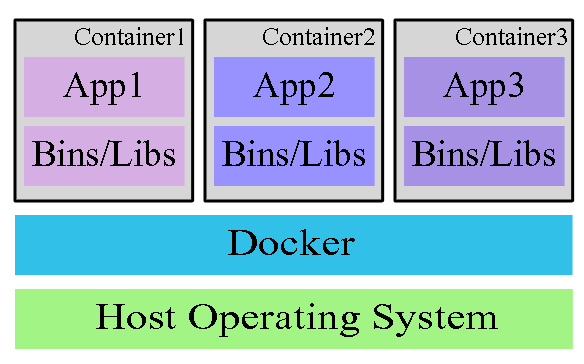
Docker runs an application in an isolated environments. Every running instance is called as container. Docker is designed to run any application with minimal operating system. Making it faster and efficient to run. We can include required library files and exclude extra things like unnecessary applications and files.
For Docker installation, You can follow these official pages to install Docker according to your preferred operating system. https://docs.docker.com/get-docker or https://docs.docker.com/get-started/#download-and-install-docker
Once docker is installed, please run docker version command to verify the setup and check docker version.
Most important terms in docker that everyone must know
Before going further you need to know few most important terminologies, which you will need almost every time you are working with docker.
What is Docker Image?
Docker image is the read-only and ready to execute binary package of application.
Docker image contains everything needed to run an application including all dependencies, configurations, scripts, libraries, etc. You can run it directly without any other setup or configuration.
To run application in docker you need to have a docker image.
We can say it is like java jar or war file.
Docker Hub
Docker hub is a repository for images. From there you can download opensource, public, community application images.
You don’t have to always create docker image, you can download available images from docker hub and use in your applications.
Free public space is also available for storing or sharing customized images. https://www.docker.com/products/docker-hub
If you know maven you can compare it to be like maven repository.
Docker Containers
Docker containers are the running instances of docker images. So when you run any docker image, new container is created. We can create multiple instances from same image.
We can compare it like a java runtime object from class file or application context.
Every container runs in an isolated environment like virtual machine.
Containers are the lightweight and better alternatives to the virtual machine, as they use the memory as per requirement and do not need complete virtual operating system.
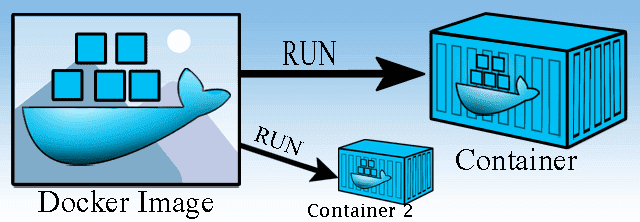
8 Most important docker commands for beginner
These are the most important commands that everyone must know. If you try to run all these commands and see the output, then it will be easier to understand them.
- Docker ps: List container details
- Docker run: Creates new container from image
- Docker exec: Execute new command into running container
- Docker images: List all downloaded images
- Docker stop: stops one or more running container with 10 seconds of default waiting time
- Docker kill: stops one or more running container forcefully
- Docker rm: delete one or more container
- Docker rmi: delete one or more downloaded image
1. Docker ps
Docker ps command is used to list containers' details. By default it lists all running containers. We can use different options to control the list.
Syntax: docker ps [OPTIONS]
For example
List all running containers: Docker ps
List all running and stopped containers: Docker ps --all
Docker ps : List all running containers
Docker ps --all : List all running and stopped containers
For more details and options for the commands visit docker docs
2. Docker run
Docker run is the most used and most powerful command. This command is used to create new docker container from docker image.
Syntax: docker run [OPTIONS] IMAGE [COMMAND] [ARG…]
This command comes with wide range of options like network setting, environment variables, port etc
Also it is possible to provide specific commands or arguments to run in container.
For example
Run ubuntu container and print current directory path
docker run ubuntu pwd
Note: --rm is optional and it is used to remove container after it is stopped
docker run --rm ubuntu pwd
Run ubuntu container and keep it connected
Docker run --rm -it ubuntu
Type exit to close the connection
3. Docker exec
The docker exec command is used to run a new command in already running container.
Syntax: docker exec [OPTIONS] CONTAINER_ID/NAME COMMAND [ARG…]
For example
We need to run this example in 2 steps, First we need to create an running container with some name like myContainer
docker run --rm -it --name myContainer ubuntu
Open and run into another shell/cmd prompt, to list files or to connect the container
docker exec myContainer ls
docker exec –it myContainer bash
4. Docker images
This command is used to list downloaded images, sometimes it is useful see all downloaded images.
Docker images
5. Docker stop
Docker stop command is used to stop one or more running container with 10 seconds of default waiting time.
syntax: docker stop container1 [containers . . ]
for example
This example we will run in 2 steps,
First let's create some running containers using following command in different command prompts,
docker run --rm -it --name myContainer1 ubuntu
docker run --rm -it --name myContainer2 ubuntu
Now open another Command prompt and check running containers then stop it.
Docker ps
docker stop myContainer1 myContainer2
6. Docker kill
Docker kill command is used to stop one or more running container forcefully.
Syntax: docker kill container1 [containers ...]
To kill all running containers in linux or windows powershell
docker kill $(docker ps -q)
To kill all running containers using windowd cmd prompt
for /F %cin ('docker ps -q') do docker kill %c
7. Docker rm
Docker rm command deletes one or more container.
Syntax: docker rm [OPTIONS] CONTAINER [CONTAINER…]
Note: to remove running container force (–f) option is required.
Example
docker rm -f myContainer1 myContainer2
Delete all stopped containers using linux bash or windows powershell
docker rm $(docker ps -a -q)
Remove all stopped containers using windowd cmd prompt
for /F %c in ('docker ps -q') do docker rm %c
8. Docker rmi
Docker rmi command is used to delete one or more downloaded images. This command helps to free up memory by deleting not required images.
Syntax: docker rmi [OPTIONS] IMAGE [IMAGE…]
example
docker rmi ubuntu
Remove all images in linux or windows powershell
docker rmi $(docker images -a -q)
Remove all images using windowd cmd prompt
for /F %c in ('docker images -aq') do docker rmi %c
Fast track reading
- Docker packages application with required files and setting then share and run directly
- Docker image is the read-only and ready to execute binary package of application which includes all dependencies, configurations, scripts, library, etc
- From Docker hub we can download open source, public, community application images
- Docker containers are the running instance of docker images
- Multiple instances can be created from same image