Super keyword in java is one of the most used and very important keyword.
In java super keyword refers immediate super(parent) class objects.
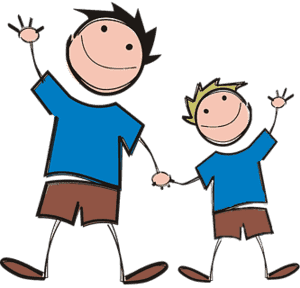
In java super keyword is only used in case of Inheritance. Whenever we create instance of class, parent class instance is also created. The parent class instance can be accessed by ' super' keyword.
So you might be wondering, if we have inheritance then we can access the parent class member directly. So why do we need super keyword?
The answer is, if we have same member in derived(child) and parent class, then there is an ambiguity for JVM. JVM can not use parent class member so it uses the child class member by default. In such a case we can use super keyword for referring the parent object members.
3 use cases of super keyword in java
Accessing parent class
- Variables
- Methods
- Constructor
Accessing parent class variables
If we have variable with same name in parent and child class, then we need to use super keyword to access parent class variable.
Lets see an example for better understanding:
class Father {
String hairColor = "Black";
}
class Son extends Father {
String hairColor = "Blonde";
public void printHairColor() {
System.out.println("Parent hair color: " + super.hairColor);
System.out.println("Child hair color: " + hairColor);
}
}
public class Tester {
public static void main(String[] args) {
Son son = new Son();
son.printHairColor();
}
}
Output:
Parent hair color: Black
Child hair color: Blonde
In the above example we can see that we are having hairColor variable in Father and Son class. So in Son class we used super.hairColor to access variable from father class.
Accessing parent class methods
If we have any method with same name in parent and child class, then we need to use super keyword to access parent class method.
Lets see an example for better understanding:
class Father {
String getHairColor(){
return "Blonde";
}
}
class Son extends Father {
String getHairColor(){
return "Blonde";
}
public void printHairColor() {
System.out.println("Parent hair color: " + super.getHairColor());
System.out.println("Child hair color: " + getHairColor());
}
}
public class Tester {
public static void main(String[] args) {
Son son = new Son();
son.printHairColor();
}
}
Output:
Parent hair color: Black
Child hair color: Blonde
In the above example we can see that we are having getHairColor() method in Father and Son class. So in child class we have used super.getHairColor() to access method from father class.
Accessing parent class constructor
Super keyword can be used to call the parent class constructor. Using super we can call default or parameterized keyword.
In java every time we create an object of a class it calls the parent class constructor even if you don't specify. This is called as constructor chaining.
If we specify which constructor (default or parameterized) from parent class to call, it will call the specified constructor of parent class. If you don't specify which constructor to call, it always calls the default constructor of parent class. And if parent class does not have default constructor, then JDK throws compile time error.
We can call the parent class default constructor using super() similarly parameterized constructor using super(arg1, arg2...).
It is important that the super() should be the first line in the child class constructor.
Lets see an example for better understanding:
class Father {
String hairColor = "Black";
public Father(String hairColor) {
this.hairColor = hairColor;
}
public void printHairColor() {
System.out.println("Hair color is : " + hairColor);
}
}
class Son extends Father {
public Son() {
super("Blonde");
}
}
public class Tester {
public static void main(String[] args) {
Son son = new Son();
son.printHairColor();
}
}
Output:
Hair color is : Blonde
In the above example we can see that we are having public Father(String hairColor) in parent class. So in child Son class we used super("Blonde") to access parent class constructor.
Fast track reading
- In java super keyword refers immediate parent class objects
- In java super keyword is only used in case of Inheritance
- Super keyword in java accesses parent class variables, methods or constructor
- Super call for the parent class constructor should be the first line in the child class constructor
- If you don't specify super() in child class, JVM will provide it implicitly