Software Design patterns are very important part of any developers life. Everyday we do many things. For instance, bag packing, cooking, building something or writing some software code. We can either do the way things come into our mind, or we can use our or experts' experiences and suggestions.
There are so many things we all do repeatedly. There are some solutions and best practice guidelines that help to remove or reduce the repetition and consequently, achieve best results.
Without design pattern Using design pattern
The best described solutions for the most common as well as repeating problems of software developers are called as design pattern.
These are the solutions obtained by experienced developers.
Most importantly, they are not a solution to a specific problem however, they are concepts. In short, design patterns are the blueprints of solutions to different types of problems.
Therefore, for any software development there are many scenarios where you can use design patterns.
Who Should use design pattern?
Design patterns are not the programming language specific like java, C# or .net. So, it does not matter which programming language you are working with. Some of these design patterns are so straight forward that we implement them in our code without even realizing it.
Why we should use design patterns?
We can develop any software application without using the design patterns. However, using design patterns add many benefits to programming.
- Provides widely accepted guidelines which are tested by experts
- Design patterns make code reusable
- More maintainable
- Speed up development process
- Reduce bugs in the code
For example, when you write some code in java and your fellow programmer later tries to add extension to your code. For this purpose he needs to understand the flow of the code. Now, if you have used java design patterns he will be able to understand easily as you have followed common solution.
What is Gang of four?
Four authors Erich Gamma, Richard Helm, Ralph Johnson and John Vlissides published book "Design Patterns: Elements of Reusable Object-Oriented Software".
They introduced design patterns for software development, consequently they were known as the Gang of four(GOF).
Types of Software design pattern:
Creational design patterns
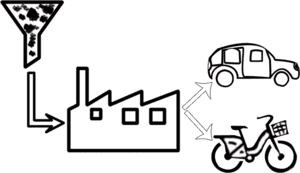
These patterns are used when we need to create new objects.
Sometimes object creation is not simple task. It could be complex process. In other words, it may involve may steps or may need more resources like time, memory or processing power. In such cases we can use Creational design patterns.
These design patterns deal with creation of classes or objects. Moreover, It helps to increase re-usability and flexibility of the code.
- Singleton : Exactly one instance is present. Therefore, entire application uses the same object
- Prototype: Copy from existing product and make changes if required
- Factory Method : Create different objects according to request
- Abstract Factory : Create different factory according to request. Also called as factory of factory
- Builder : Helps to create object step by step
- Object Pool : Reuse the object which are not in use any more. Consequently, avoid creation of very expensive objects
- Dependency Injection pattern: Get object from injector service, who is responsible for creating objects.
- Lazy initialization pattern: At the time of first use object is created
Structural design pattern
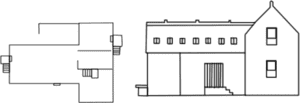
Structural design patterns are used to design the class structure and architecture.
We can use these for creating new functionality or extending the existing functionality.
Many times we come across a scenario where we have incompatible objects.For instance, consider we have mobile and charger of different companies. As a result, we can not connect them because they are not compatible.
Likewise, assume that we have java code for MySQL database but we have Oracle database. In such cases we need to create some small project. This project will help both of them communicate. This solution can be called as Java Structural design pattern implementation.
- Adapter: Provides the solution for helping incompatible things to communicate with each other
- Bridge: Separates things so that they can work independently as well
- Composite: Allows to operate objects in a tree structure hierarchy
- Decorator: Allows to add new functionality to existing object
- Facade: Provide the simple interface to use many small systems. Additionally, hides the complexity by wrapping the different process. We can use without knowing each process
- Flyweight: Allows to reuse the object if available otherwise create new and store in memory
- Proxy: Represents the functionality of other object. Protect original object from direct access to prevent damage
Behavioral design patterns
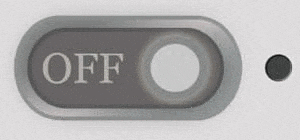
Behavioral design patterns are used to define class behavior.
In most of the cases we have seen that somethings are dependent on others. For instance, when we do some online payments we get notifications. Similarly, when you need tickets you first do the payment then you get tickets.
To sum it up, Behavioral design patterns deal with the communication between the objects, and behavior of the object is dependent on the another
- Chain of responsibility: Create chain of processes and execute one after another consequently for the request
- Command: Convert each request into individual command or action for execution
- Interpreter: Evaluates the meaning of expression
- Iterator: Accesses sequential elements from the group of elements
- Mediator: Provide intermediate solution for communicating the objects. Additionally, This reduces the complexity. Using this two classes don’t communicate directly
- Memento: Keeps track of previous states. This help to restore to any state if we need
- Observer: Keeps observing the target . Takes the action if state of target changes
- State: Different behavior according to different states
- Strategy: Behavior is dependent on the environment /context or input
- Template: Create structure/format, reused by many classes
Fast track reading
- Best described solution for the most common and repeating problems of software developers are called as design patterns.
- Firstly, they are not a solution to a specific problem
- Secondly, Design patterns are not programming language specific
- In software development there are many scenarios where you can use design patterns
- Additionally, solutions are provided and accepted and tested by experts
- Originally introduced by Gang of four (GoF)
- 3 main categories, Creation pattern, Structural pattern and behavioral pattern
Design patterns is something I’ve ignored quite for a while.. Guess, I need to get my feet wet. Interesting read.
Thank you very much for taking time to read and appreciate the efforts. This motivates us to keep up our work!! Stay tuned for more such interesting blogs. Hope you like them as well!!
Really nice blog. explained in easy language so got interest in reading!
I am happy to hear that i am being able to help other developers. I am trying my best to make it as easy and simple as possible. There are more blogs coming on Design patterns like learn adapter design pattern in java. Please stay connected and let us know what you feel about the post.
This blog Design patterns in Software development helps me
a lot with my woodworking projects.